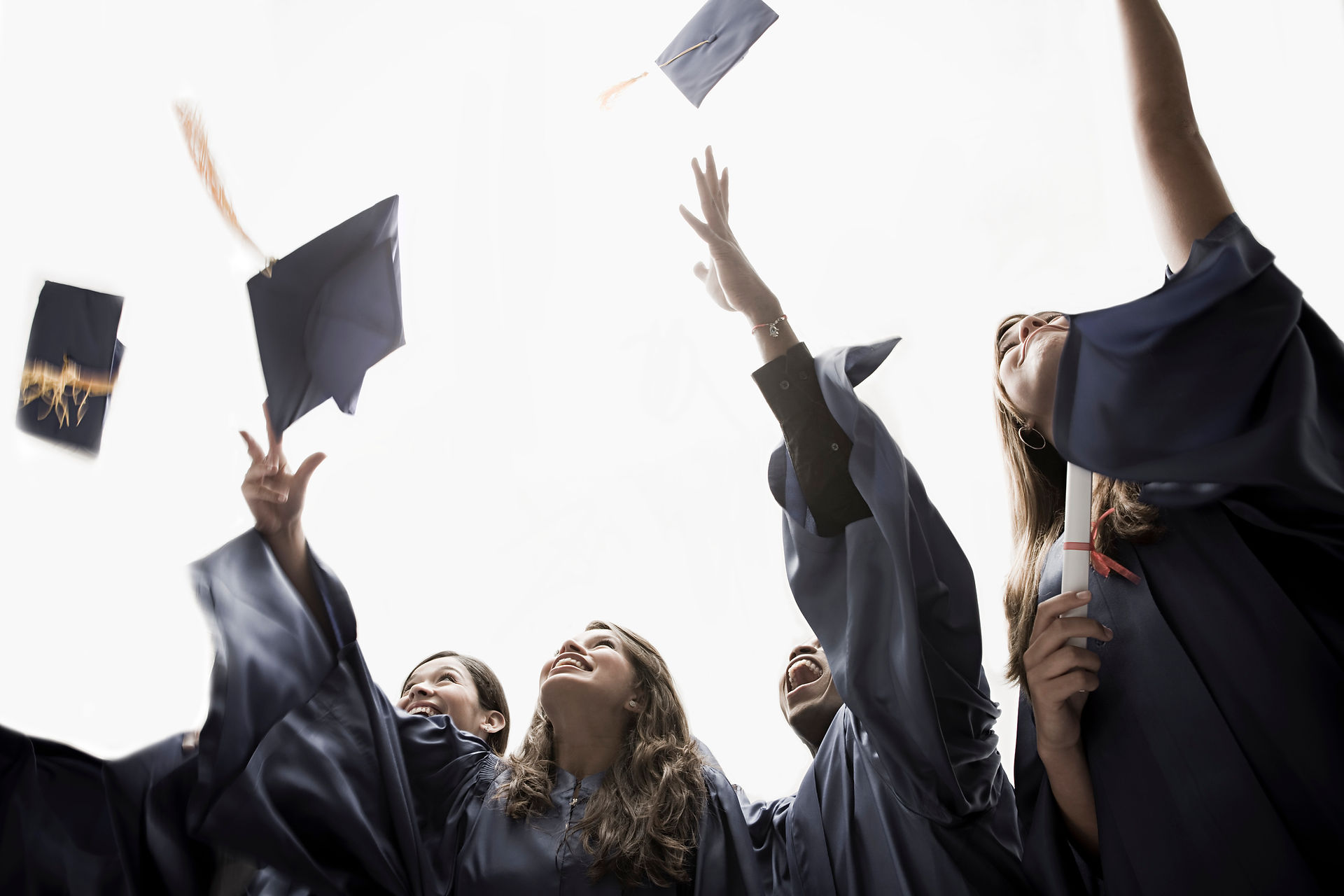
Get through the course of
"Java Essentials"
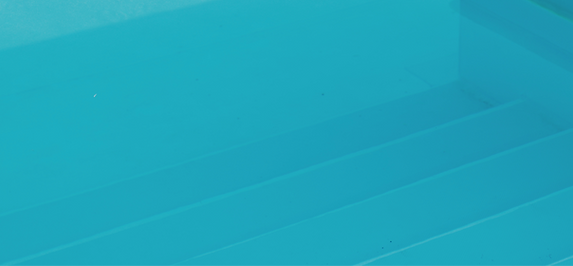
Week 1: Introduction to Java Programming Day 1: Course Introduction & Setting Up the Environment Overview of Java programming Installing JDK and setting up IDE (e.g., IntelliJ IDEA, Eclipse) Writing and executing your first Java program Overview of Java syntax and structure Day 2: Data Types and Variables Primitive data types (int, float, char, boolean, etc.) Declaring and initializing variables Typecasting and type conversion Constants and literals Day 3: Operators and Expressions Arithmetic, relational, logical, and bitwise operators Assignment and comparison operators Expression evaluation and precedence Introduction to Math class for advanced math operations Day 4: Control Structures - Conditional Statements If-else statements Switch-case structure Nested conditions and decision-making Day 5: Control Structures - Loops While loop, do-while loop For loop, enhanced for loop Nested loops and loop control (break, continue) Day 6: Arrays Declaring and initializing arrays Single-dimensional and multi-dimensional arrays Common array operations (traversal, searching, sorting) Day 7: Methods and Functions Defining methods Method parameters and return values Method overloading Recursion basics
Week 2: Object-Oriented Programming (OOP) Day 8: Introduction to OOP Concepts Object and Class fundamentals Understanding attributes and behaviors The new keyword and creating objects Real-world analogies of objects and classes Day 9: Classes and Objects in Java Class definition Constructors (default, parameterized) Class vs object methods Memory allocation for objects Day 10: Encapsulation Access modifiers: public, private, protected, and default Getters and Setters Encapsulation and data hiding in Java Day 11: Inheritance Basics of inheritance and its advantages extends keyword Overriding methods Using super keyword Day 12: Polymorphism Compile-time vs runtime polymorphism Method overloading vs method overriding Dynamic method dispatch Day 13: Abstract Classes and Interfaces Defining abstract classes and methods Interfaces in Java and their implementation Differences between abstract classes and interfaces Day 14: Review and Hands-On Practice Recap of OOP concepts with exercises Solving real-world problems using OOP principles Q&A session
Week 3: Advanced Java Concepts Day 15: Exception Handling Introduction to exceptions and error types Try-catch block and multiple catch Throw, throws, and finally Creating custom exceptions Day 16: File Handling Working with files using File class Reading and writing files using FileReader and FileWriter Buffered reader/writer for efficient file handling Exception handling in file operations Day 17: Collections Framework (Part 1) Overview of collections framework List interface and ArrayList class Iterating through collections Introduction to LinkedList Day 18: Collections Framework (Part 2) Set interface and HashSet class TreeSet and SortedSet Map interface: HashMap and TreeMap Day 19: Working with Strings Creating and manipulating strings String methods (charAt, substring, indexOf, etc.) StringBuilder and StringBuffer classes for mutability Day 20: Multithreading (Part 1) Introduction to threads and processes Creating threads using Thread class and Runnable interface Thread lifecycle and states Day 21: Multithreading (Part 2) Synchronization in multithreading Deadlock and thread communication Executor framework
Week 4: Final Topics and Project Work Day 22: Java I/O Streams Introduction to Input/Output Streams Working with Byte Streams and Character Streams Data Streams and Object Streams Day 23: Networking in Java Basics of networking (TCP, UDP) Socket programming Creating client-server applications Day 24: Introduction to Java GUI (Swing) Basics of GUI development Components like buttons, labels, text fields Event handling in Java Day 25: JavaFX for Modern UI Introduction to JavaFX framework Creating simple graphical applications Layouts and UI controls Day 26: JDBC (Java Database Connectivity) Introduction to databases and SQL Setting up JDBC with MySQL or PostgreSQL Connecting and querying a database using JDBC Day 27: Maven and Build Tools Introduction to Maven and build tools Setting up and configuring Maven projects Understanding POM and dependencies Day 28: Debugging and Testing in Java Introduction to debugging techniques Unit testing with JUnit Using IDEs for debugging and testing Day 29: Mini Project (Part 1) Choose a mini-project that incorporates all learned concepts Plan and design the project structure Day 30: Mini Project (Part 2) and Course Conclusion Code implementation of the mini-project Final Q&A and course wrap-up Feedback and certification distribution